택시 요금계 만들기
[MY CODE]
CAR CODE
public class Car {
String carName;
String color;
String maker;
int speed;
public Car() {
this("쏘나타","검정색","현대");
}
public Car(String color) {
this("쏘나타",color,"현대");
}
public Car(String carName,String color) {
this(carName,color,"현대");
}
public Car(String carName,String color, String maker) {
//super(); //생략가능.클래스 상속하기 위해선 반드시 메모리 올라가야함.
//메모리 올라가는 시점은 생성자 호출되는 시점. new할때!
//모든 클래스의 최상위 클래스는 object 이고 그것을 호출하는 것이 super이다.
this.carName = carName;
this.color = color; this.maker = maker;
}
int speedUp(int speed) {
this.speed += speed;
return this.speed;
}
int speedDown(int speed) {
this.speed -= speed;
if (this.speed <0) stop();
return this.speed;
}
void stop() {
speed= 0;
}
String info() {
return maker + "에서 만든 " + color + " " + carName;
}
}
TAXI CODE
int totalPrice;
int price;
int initHour;
int sumHour;
public Taxi() {
//super(); //생략가능. 상위클래스 생성자 중에서 default 값 불러옴
// this와 super는 코드 맨윗줄에 있어야함.
// Object > Car > Taxi 순으로 메모리에 만들어짐.
//Taxi에서 super 불러오면 Car 의 super 불러지고 car의 상위 class는 default이기 때문에
최종적으로 object 불러와짐.
//그 후 object 메모리에 올라가고 car 올라가고 taxi 올라감.
basicPrice = 3800;
}
public Taxi(String carName,String color, String maker) {
//상속은 생성자 상속X되기 때문에 Taxi에 Car과 같은 String3개짜리 만들어라.
super(carName,color,maker); //상위클래스의 이름,색,maker에 내가 지정한것 넣음.
basicPrice = 3800;
}
int initPrice() {
// 시작버튼
Calendar calendar = Calendar.getInstance();
initHour = calendar.get(Calendar.HOUR_OF_DAY); // 시작했을 때 시간
return initHour;
}
int sumPrice(int km){
if (initHour >=0 && initHour<=6){ // 시작 시간이 0~6시면
basicPrice = 5000; //기본요금 5000
calcPrice(km); //calcPrice 메소드 돌림.
price return받음 price +=50*km; //price에 50원/km 더함.
totalPrice+=50*km;
} else { //일반요금.
calcPrice(km);
}
return price; //return price
}
int calcPrice(int km) {
price = basicPrice + 100 * km;
totalPrice += price;
return price;
}
}
TAXIGUEST CODE
public class TaxiGuest {
public static void main(String[] args) {
Taxi t1 = new Taxi();
System. out. println("t1 >> " + t1.info());
//Taxi t2 = new Taxi("K5","감홍색","기아");
//System. out. println("t1 >> " + t1.info());//에러남. 생성자는 상속X되기 때문, 따라서 Taxi에 string
3개짜리 생성자 만들어줘야함.
Taxi t2 = new Taxi("K5","감홍색","기아");
System. out. println("t1 >> " + t1.info());
int km = 20;
System. out. println(t2.info() + "를 타고 구디에서 강남까지 " + km + "km이동!!!");
int initHour = t2.initPrice();
int price = t2.sumPrice(km);
System. out. println("요금 : " + price + "원");
System. out. println("누적 수익 : " + t2.totalPrice + "원");
km = 30;
System. out. println(t2.info() + "를 타고 강남에서 구디까지 " + km + "km이동!!!");
initHour = t2.initPrice();
price = t2.sumPrice(km);
System. out. println("요금 : " + price + "원");
System. out. println("누적 수익 : " + t2.totalPrice + "원");
}
}
[선생님 코드]
Taxi code
import java.util.*;
public class Taxi extends Car {
int basicPrice;
int totalPrice;
int price;
int pricePerKm;
public Taxi() {
super();
}
public Taxi(String carName, String color, String maker) {
super(carName, color, maker);
}
void initPrice() {
Calendar calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR_OF_DAY);
if(hour < 6) {
basicPrice = 5000;
pricePerKm = 150;
} else {
basicPrice = 3800;
pricePerKm = 100;
}
}
int calcPrice(int km) {
price = basicPrice + (km * pricePerKm);
return price;
}
void sumPrice() {
totalPrice += price;
price = 0;
basicPrice = 0;
}
}
TaxiGuest code
public class TaxiGuest {
public static void main(String[] args) {
Taxi t1 = new Taxi();
System.out.println("t1 >> " + t1.info() + " 기본요금 : " + t1.basicPrice);
Taxi t2 = new Taxi("K5", "감홍색", "기아");
System.out.println("t2 >> " + t2.info() + " 기본요금 : " + t2.basicPrice);
int km = 20;
t2.initPrice();
System.out.println(t2.info() + "를 타고 구디에서 강남까지 " + km + "km이동!!!");
int price = t2.calcPrice(km);
t2.sumPrice();
System.out.println("요금 : " + price + "원");
System.out.println("누적 수익 : " + t2.totalPrice + "원");
km = 20;
t2.initPrice();
System.out.println(t2.info() + "를 타고 강남에서 구디까지 " + km + "km이동!!!");
price = t2.calcPrice(km); t2.sumPrice(); System.out.println("요금 : " + price + "원");
System.out.println("누적 수익 : " + t2.totalPrice + "원");
}
}
[결과]
할증 붙음.
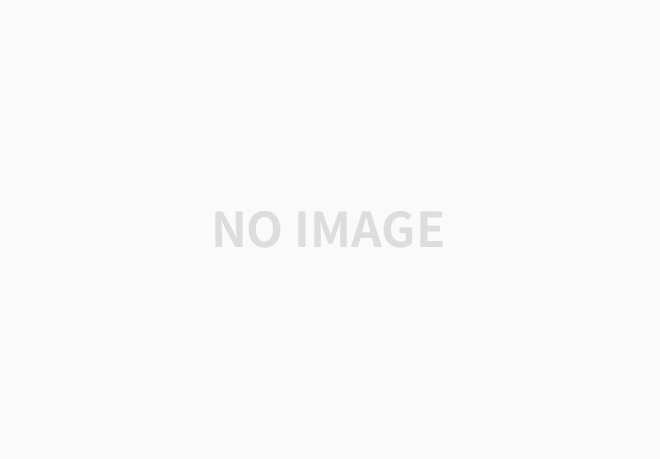
[출처] 빡쏘끼룩
'IT > JAVA' 카테고리의 다른 글
[Java]상속 (inheritance) - 다형성 (0) | 2020.07.22 |
---|---|
[Java]Override 2 (0) | 2020.07.21 |
[Java]상속 (inheritance)/overriding(재정의)/super (0) | 2020.07.18 |
[Java]스타크래프트 마린 만들기/call by reference, call by value (0) | 2020.07.17 |
[Java]사각형과 원 넓이, 둘레 구하기 code (0) | 2020.07.08 |